Mastering Web App Architecture: Insights, Best Practices, and Real-World Examples
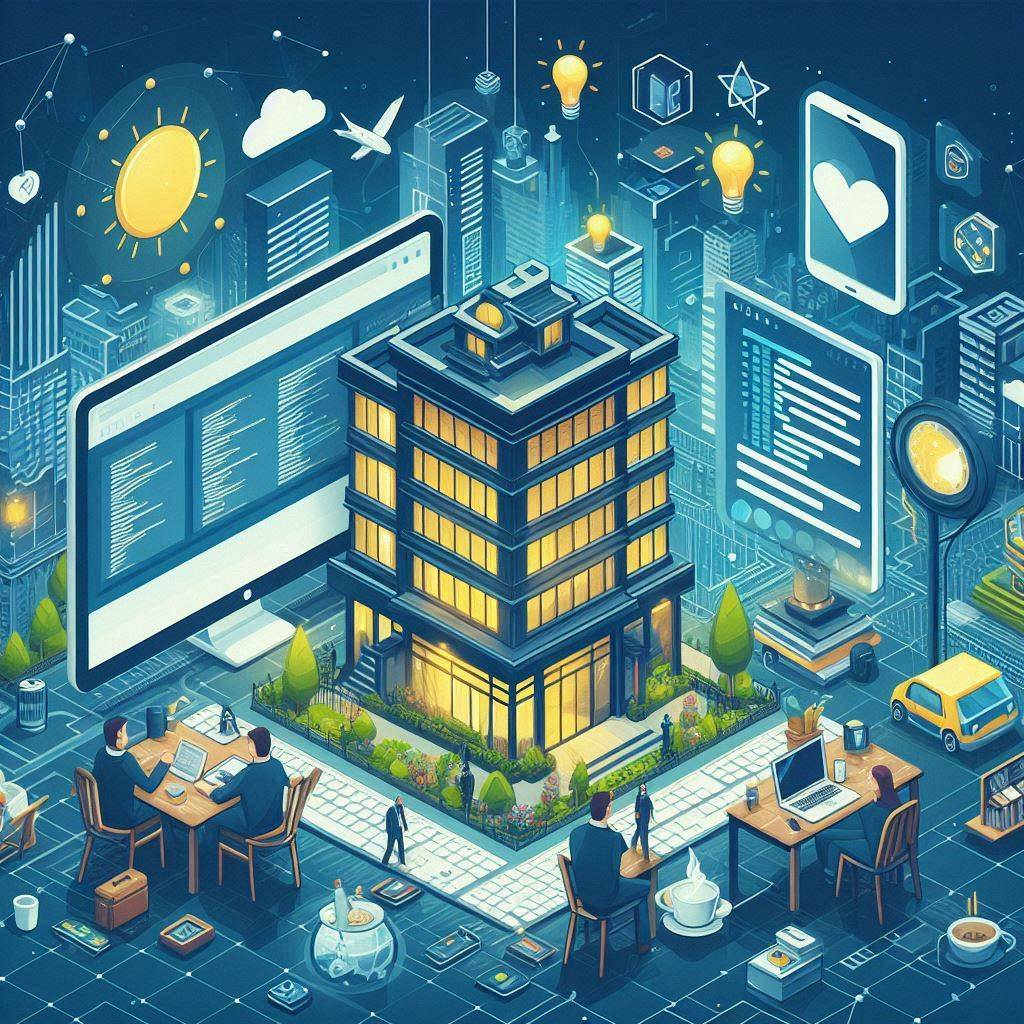
Web app architecture is crucial in building scalable, maintainable, and efficient applications. This blog delves into the intricacies of web app architecture, exploring different styles, technologies, best practices, and real-world examples to provide a comprehensive understanding.
Types of Web App Architectures
- Monolithic Architecture
- Definition: A single, unified codebase where all components are interconnected.
- Use Case: Ideal for small projects with limited functionality.
- Example: Early versions of eBay used a monolithic architecture, which later became challenging to scale and maintain.
- Microservices Architecture
- Definition: Divides an application into independent services, each responsible for a specific functionality.
- Use Case: Suitable for large, complex applications requiring scalability.
- Example: Netflix uses microservices to manage its vast array of functionalities, allowing individual services to scale independently.
- Serverless Architecture
- Definition: Leverages cloud services to handle server operations dynamically.
- Use Case: Ideal for applications with fluctuating traffic and usage patterns.
- Example: Coca-Cola’s vending machine backend operates on a serverless architecture, efficiently handling spikes in usage.
Front-End Technologies
Front-end architecture focuses on the user interface and experience. Key technologies include:
- HTML/CSS: The backbone of web page structure and styling.
- JavaScript Frameworks:
- React: Efficiently manages complex UI updates. Used by Facebook.
- Angular: Provides a robust framework for dynamic single-page applications (SPAs). Employed by Google.
- Vue.js: Simplifies building interactive UIs and SPAs. Adopted by Alibaba.
Back-End Technologies
The back-end architecture handles data processing and business logic. Prominent technologies include:
- Node.js: Enables server-side JavaScript execution.
- Example: LinkedIn uses Node.js for its mobile backend to handle numerous connections efficiently.
- Python/Django: Offers rapid development and a clean design.
- Example: Instagram’s backend runs on Django, allowing quick feature deployments.
- Ruby on Rails: Favors convention over configuration.
- Example: GitHub uses Rails for its web interface.
Database Management
Choosing the right database is vital for performance and scalability:
- Relational Databases (SQL):
- MySQL: Preferred for structured data.
- Example: Used by WordPress to manage content efficiently.
- PostgreSQL: Known for its robustness and scalability.
- Example: Employed by Skype for its real-time messaging infrastructure.
- NoSQL Databases:
- MongoDB: Suitable for unstructured data and high availability.
- Example: Used by Uber to store rider and driver information.
- Cassandra: Handles massive amounts of data across multiple servers.
- Example: Facebook uses Cassandra for its messaging platform.
API Design
APIs are critical for front-end and back-end communication:
- RESTful APIs: Standard for creating scalable web services.
- Example: Twitter’s API allows third-party developers to integrate its functionalities into their applications.
- GraphQL: Enables clients to request specific data.
- Example: GitHub’s API uses GraphQL to provide precise data queries, reducing data transfer.
Security Best Practices
Ensuring security in web app architecture is paramount:
- Authentication and Authorization: Implement robust systems like OAuth and JWT.
- Example: Google’s OAuth 2.0 allows secure user authentication.
- Data Encryption: Use HTTPS and encrypt sensitive data.
- Example: Online banking platforms like Chase use HTTPS for secure transactions.
- Regular Updates and Patching: Keep all components updated.
- Example: Adobe regularly updates its software to patch vulnerabilities.
Performance Optimization
Optimizing performance ensures a smooth user experience:
- Caching: Store frequently accessed data to reduce server load.
- Example: Content Delivery Networks (CDNs) like Cloudflare cache content to reduce latency.
- Load Balancing: Distribute traffic across multiple servers.
- Example: Amazon uses Elastic Load Balancing to manage its vast traffic.
- Asynchronous Processing: Handle background tasks efficiently.
- Example: LinkedIn processes notifications asynchronously to improve user experience.
DevOps and CI/CD
Integrating development and operations enhances deployment:
- Continuous Integration (CI): Regularly merge code changes.
- Example: Facebook uses CI to deploy updates several times a day.
- Continuous Deployment (CD): Automate the release process.
- Example: Amazon deploys updates every 11.6 seconds using CD.
Real-World Architecture Examples
- Netflix
- Architecture: Microservices
- Details: Netflix’s architecture allows it to deliver streaming services to millions of users globally. Each service is designed to handle a specific task such as user authentication, recommendation engine, or video encoding. This microservices approach enables Netflix to deploy updates rapidly without affecting the entire system.
- Amazon
- Architecture: Microservices and Serverless
- Details: Amazon’s e-commerce platform relies heavily on microservices to manage various functionalities like product search, order processing, and payment handling. Additionally, Amazon uses serverless architecture for event-driven tasks, ensuring scalability during peak shopping seasons.
- Facebook
- Architecture: Monolithic to Microservices
- Details: Initially, Facebook used a monolithic architecture, but as the user base grew, it transitioned to a microservices architecture. This change helped Facebook manage vast amounts of data, enabling features like real-time chat, notifications, and content delivery efficiently.
- Uber
- Architecture: Microservices
- Details: Uber uses a microservices architecture to handle its complex logistics network. Each service manages a specific aspect of the business, such as ride matching, fare calculation, and driver dispatching. This architecture allows Uber to scale services independently and introduce new features without disrupting the entire system.
- Spotify
- Architecture: Microservices
- Details: Spotify’s microservices architecture supports its music streaming platform, with services dedicated to tasks like music recommendations, playlist management, and user data analysis. This setup allows Spotify to offer personalized experiences and maintain high performance even with millions of concurrent users.