Best Practices for iOS Development with SwiftUI
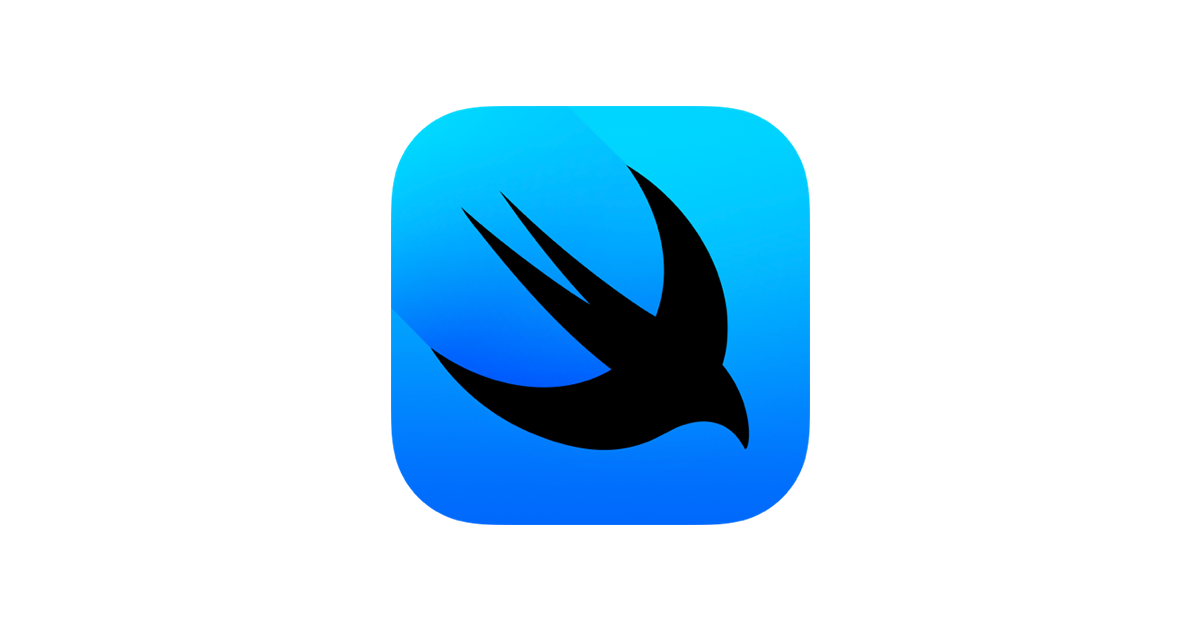
Leveraging these advanced techniques can significantly enhance the performance, maintainability, and scalability of your applications. Drawing from our extensive experience, here are some advanced best practices for mastering SwiftUI.
1. Architectural Patterns: MVVM and Combine
Model-View-ViewModel (MVVM) Architecture
- Separation of Concerns: MVVM separates the user interface (View) from the business logic (ViewModel) and data (Model). This separation promotes a clean architecture and makes code easier to test and maintain.
Implementation: Use Swift’s @Published
properties within your ViewModel to automatically update the UI when data changes.
class UserViewModel: ObservableObject {
@Published var username: String = ""
@Published var password: String = ""
func login() {
// Perform login action
}
}
struct LoginView: View {
@ObservedObject var viewModel = UserViewModel()
var body: some View {
VStack {
TextField("Username", text: $viewModel.username)
SecureField("Password", text: $viewModel.password)
Button("Login") {
viewModel.login()
}
}
.padding()
}
}
Combine Framework
- Reactive Programming: Combine provides a declarative Swift API for processing values over time, allowing for sophisticated data flow handling and asynchronous event handling.
Integration: Combine integrates seamlessly with SwiftUI. Use @Published
properties in your ViewModel and bind them to your views.
import Combine
class DataFetcher: ObservableObject {
@Published var data: [String] = []
private var cancellable: AnyCancellable?
func fetchData() {
cancellable = URLSession.shared.dataTaskPublisher(for: URL(string: "https://api.example.com/data")!)
.map { $0.data }
.decode(type: [String].self, decoder: JSONDecoder())
.replaceError(with: [])
.receive(on: DispatchQueue.main)
.assign(to: \.data, on: self)
}
}
2. Performance Optimization
Lazy Loading for Improved Performance
Lazy Stacks and Grids: Use LazyVStack
, LazyHStack
, and LazyGrid
to load views on-demand, improving performance for large data sets.
struct ContentView: View {
let items = Array(1...1000)
var body: some View {
ScrollView {
LazyVStack {
ForEach(items, id: \.self) { item in
Text("Item \(item)")
}
}
}
}
}
Minimize View Updates
Avoid Unnecessary State Changes: Only update views when absolutely necessary. Using computed properties wisely can help avoid unnecessary re-renders.
struct ContentView: View {
@State private var counter = 0
var body: some View {
VStack {
Text("Counter: \(counter)")
Button("Increment") {
counter += 1
}
}
}
}
View Builder for Complex Views
Custom View Builders: Create custom view builders for complex views to enhance code readability and reusability.
struct CustomCard<Content: View>: View {
var content: Content
init(@ViewBuilder content: () -> Content) {
self.content = content()
}
var body: some View {
VStack {
content
}
.padding()
.background(Color.gray)
.cornerRadius(10)
}
}
struct ContentView: View {
var body: some View {
CustomCard {
Text("Title")
Text("Subtitle")
}
}
}
3. Advanced Animations
Custom Animations with Matched Geometry Effect
Seamless Transitions: Use matchedGeometryEffect
to create seamless animations between views.
struct ContentView: View {
@Namespace private var animation
@State private var isExpanded = false
var body: some View {
VStack {
if isExpanded {
RoundedRectangle(cornerRadius: 25)
.fill(Color.blue)
.frame(width: 300, height: 300)
.matchedGeometryEffect(id: "rectangle", in: animation)
} else {
RoundedRectangle(cornerRadius: 25)
.fill(Color.blue)
.frame(width: 100, height: 100)
.matchedGeometryEffect(id: "rectangle", in: animation)
}
}
.onTapGesture {
withAnimation {
isExpanded.toggle()
}
}
}
}
Animations with Timing Curves and Delays
Fine-tuned Animations: Customize animations using timing curves and delays to create more natural and engaging UI interactions.
struct AnimatedView: View {
@State private var isVisible = false
var body: some View {
VStack {
Text("Hello, World!")
.opacity(isVisible ? 1 : 0)
.animation(Animation.easeInOut(duration: 1).delay(0.5))
Button("Toggle") {
isVisible.toggle()
}
}
}
}
4. Accessibility and Localization
Dynamic Type and Accessibility Support
Scalable Text: Use font(.largeTitle)
and other scalable text modifiers to support dynamic type. Also, leverage SwiftUI’s accessibility modifiers to enhance accessibility.
struct ContentView: View {
var body: some View {
VStack {
Text("Welcome")
.font(.largeTitle)
.accessibilityAddTraits(.isHeader)
Button("Learn More") {
// Action
}
.accessibilityLabel("Learn more about this app")
}
}
}
Localization
Localized Strings: Use LocalizedStringKey
for internationalization and localization, ensuring your app is accessible to a global audience.
struct ContentView: View {
var body: some View {
Text("welcome_message")
}
}
Conclusion
SwiftUI offers a modern approach to building iOS applications, and mastering advanced techniques can significantly enhance your development workflow. By adopting architectural patterns like MVVM and Combine, optimizing performance with lazy loading and minimizing view updates, creating custom animations, and ensuring accessibility and localization, you can build robust, maintainable, and user-friendly applications. These advanced practices, backed by our team's extensive experience, will help you leverage SwiftUI to its fullest potential, ensuring your apps stand out in the competitive market.